Avatar A
Avatar image, profile picture.
Preview
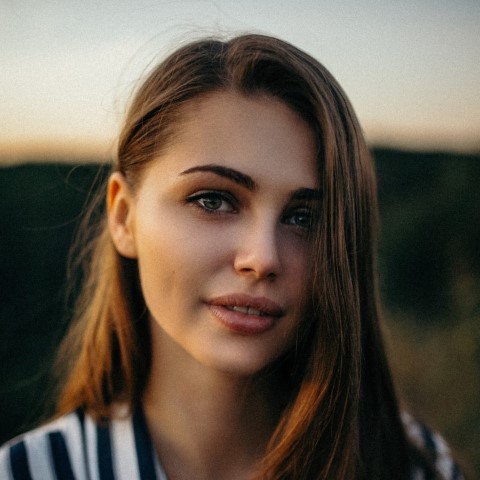
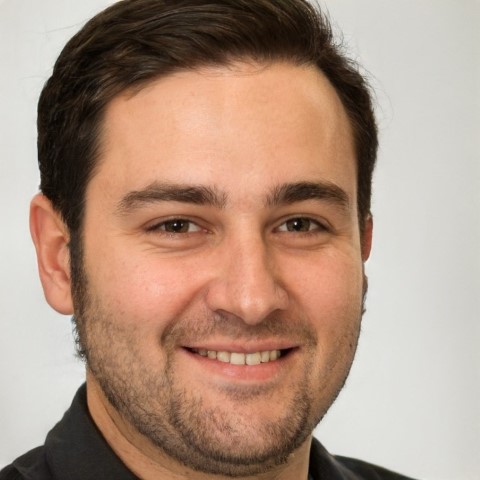
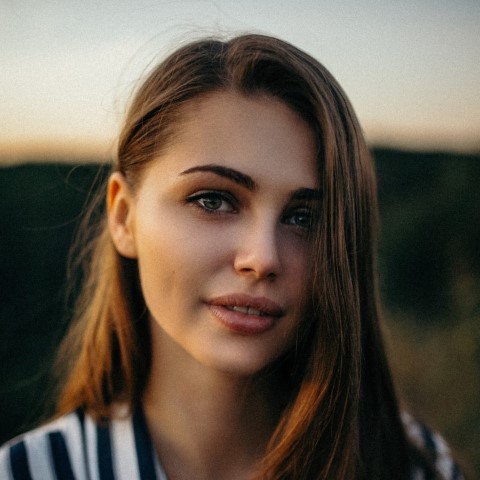
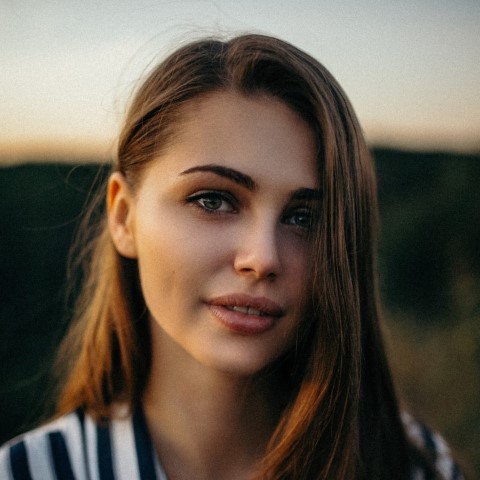
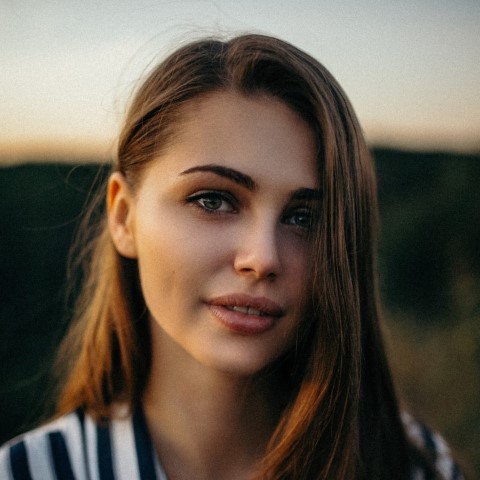
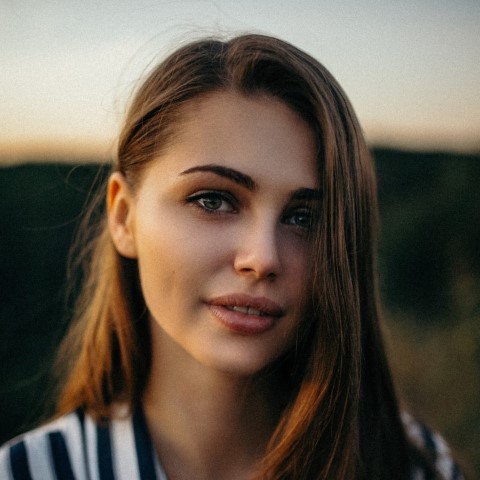
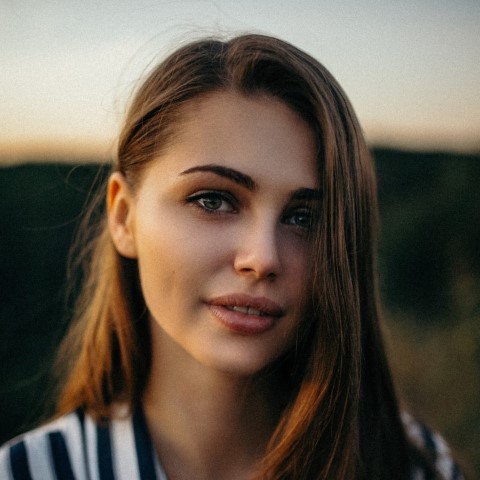
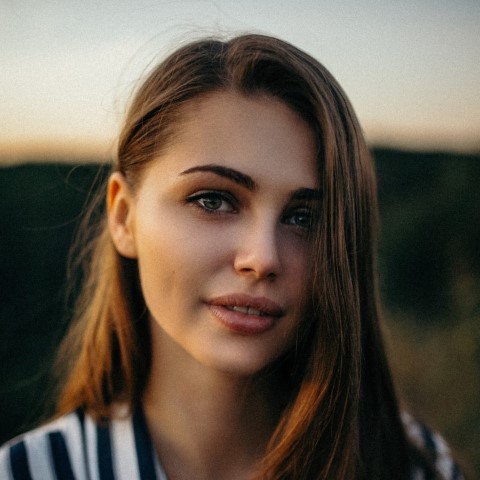
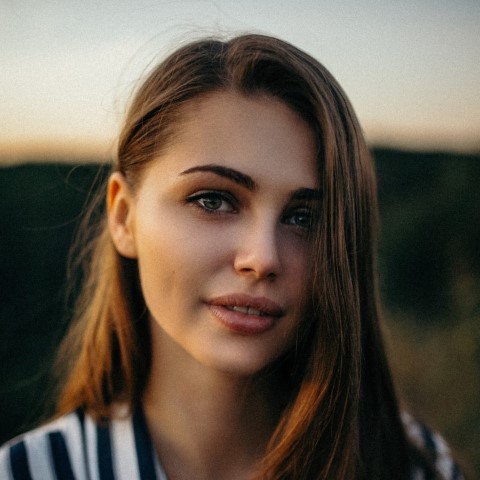
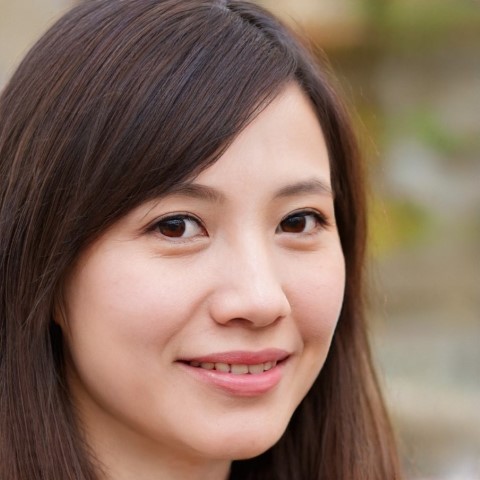
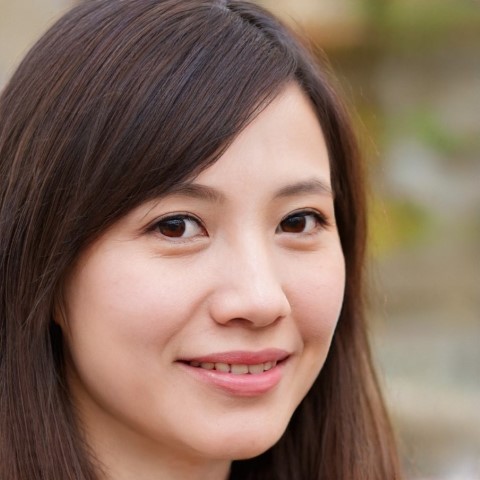
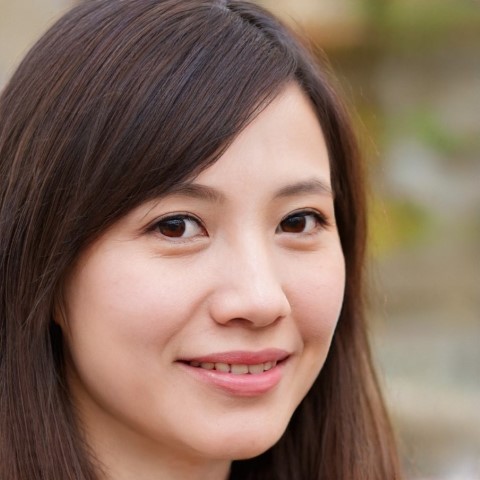
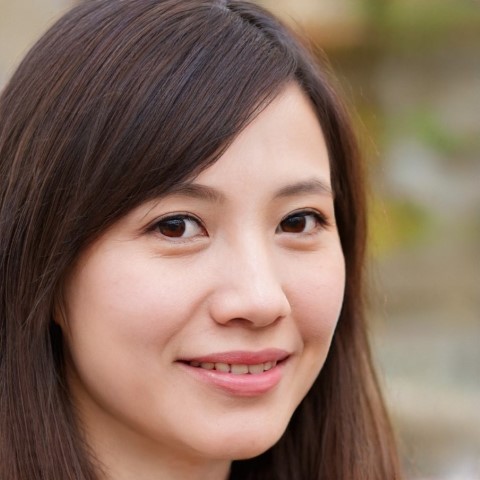
Code
import styles from "./avatar-a.module.css"; type Props = { className?: string; children?: React.ReactNode; imageSrc?: string; title?: string; size?: "xx-small" | "x-small" | "small" | "medium" | "large" | "x-large" | "full"; rounded?: "none" | "small" | "medium" | "large" | "full"; // Border borderSize?: "none" | "small" | "medium" | "large"; borderPosition?: "inside" | "outside" | "aureole"; borderColor?: "white" | "black" | "green" | "gradient"; // Label labelColor?: "none" | "green" | "red"; labelElement?: React.ReactNode; labelPosition?: "top-right" | "top-left" | "bottom-right" | "bottom-left"; labelSize?: "x-small" | "small" | "medium" | "large"; // Customized color variant?: "default" | "blue" | "orange"; }; export default function AvatarA({ className, children, imageSrc, title, size = "medium", rounded = "full", borderColor, borderPosition, borderSize = "none", labelColor, labelElement, labelSize = "medium", labelPosition = "bottom-right", variant, }: Props) { return ( <div className={[ className, styles["wrapper"], styles[`s-${size}`], styles[`r-${rounded}`], styles[`c-${variant}`], styles[`bdc-${borderColor}`], styles[`bdp-${borderPosition}`], styles[`bds-${borderSize}`], ].join(" ")} title={title} > <div className={styles["avatar"]}> {/* You may need to replace the a tag with a Link if you use Next.js */} {/* If you use Next.js, replace 'img' with 'Image' element */} {imageSrc && ( <img className={styles["image"]} src={imageSrc} alt={title?.valueOf()?.toString()} width={360} height={360} loading="lazy" /> )} {/* Optional content */} {children && <div className={styles["content"]}>{children}</div>} {(labelColor || labelElement) && ( <div className={[ styles["label"], styles[`lc-${labelColor}`], styles[`lp-${labelPosition}`], styles[`ls-${labelSize}`], ].join(" ")} > {labelElement} </div> )} </div> </div> ); }
Design
Figma design file:
Documentation
Properties
Props of the component:
-
className
(string): Specifies the CSS class of the component. -
children
(ReactNode): React children of the component. Specifies the ReactNode placed inside the component. -
imageSrc
(string): Specifies the URL of the image. -
title
(string or ReactNode): Specifies the text or element that will be used as the title. -
size
("x-small" | "small" | "medium" | "large" | "x-large" | "full"): Specifies the size of the component. -
rounded
("none" | "small" | "medium" | "large" | "full"): Specifies the border radius of the frame. -
borderSize
("none" | "small" | "medium"): Specifies the border stroke. -
borderColor
("white" | "black" | "green" | "gradient" or a customized value): Specifies the border color. -
imageRounded
("default" | "none" | "small" | "medium" | "large"): Specifies the border radius of the image. -
labelColor
("none" | "green" | "red"): Specifies the color of the label. -
labelElement
(ReactNode): Specifies the child element of the label. -
labelPosition
("top-right" | "top-left" | "bottom-right" | "bottom-left"): Specifies position of the label, placed at the bottom right by default. -
labelSize
("xx-small" | "x-small" | "small" | "medium" | "large"): Specifies size of the label. -
variant
("default" | "blue" | "orange" or a customized value): Specifies the color or theme variant of the component. See the "Sample CSS customization" below for an example of usage.
Sample CSS customization
.c-blue { --bg-color: #95beff; --fg-color: #2962b8; }